The world of static sites is evolving beyond HTML, CSS, and sometimes JS, and the advancements in static site generators have greatly contributed to this.
Author: Thom Krupa@thomkrupa November 18, 2020
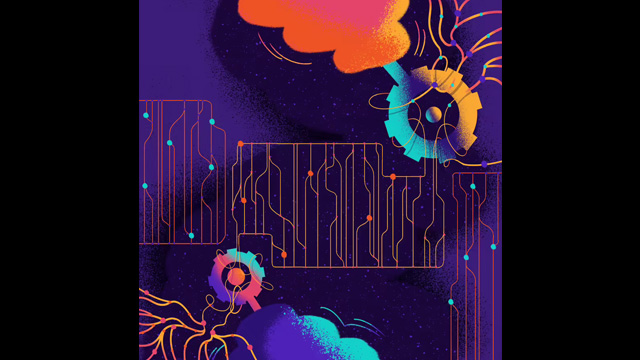
The rapidly evolving Jamstack ecosystem and static site generators are attracting significant interest in their approaches and tools. While they are not yet mainstream, it is certain that the competition among VCs to invest in companies in this field will pave the way for wider adoption.
The advantages of using a static site generator include numerous benefits, primarily speed, security, and scalability. Many web developers are adding dynamic elements using third-party APIs to enhance the functionality of static sites.
First, I will explain the basics.
1. What is a static site generator?
A Static Site Generator (SSG) is a tool for building static pages from input files. It ingests content (such as headless CMS) and applies selected templates to generate static HTML pages.
The biggest difference between the SSG approach and the classic web development approach represented by WordPress is that, instead of building pages as needed every time a user accesses the site, SSG builds pages at the time of construction. Essentially, the already built pages are stored on a CDN and provided when users access the website.
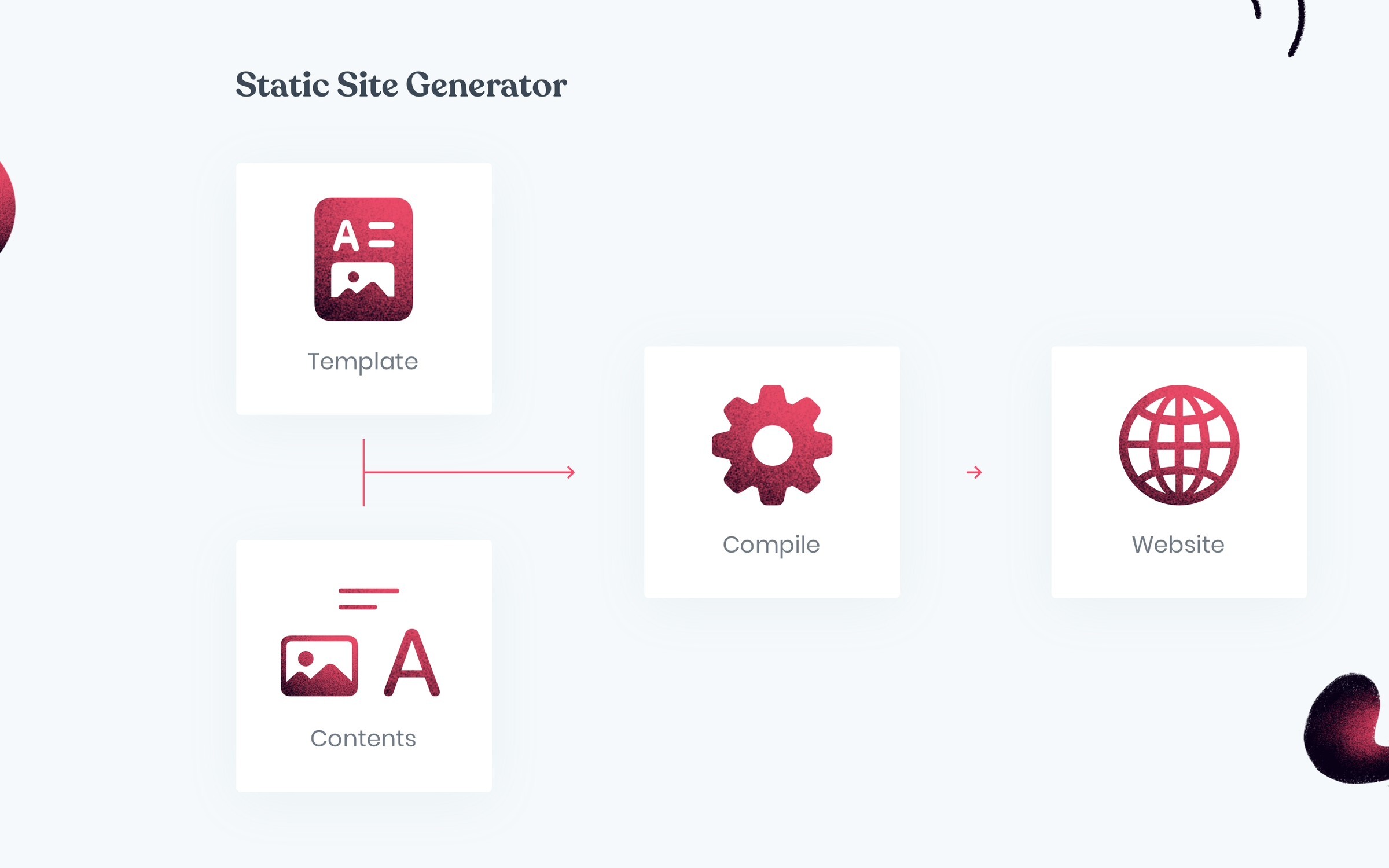
While this method allows for fast loading, it may lack the dynamic features required by current websites. However, we will discuss that later.
1-1 Why use a static site generator?
As mentioned earlier, websites created using this method have many advantages. Since they provide static HTML pages, the loading speed and overall performance of the website are significantly improved compared to traditional dynamic websites (such as those built with conventional CMS like WordPress or Drupal). Additionally, deploying or storing static sites on a server becomes remarkably easy.
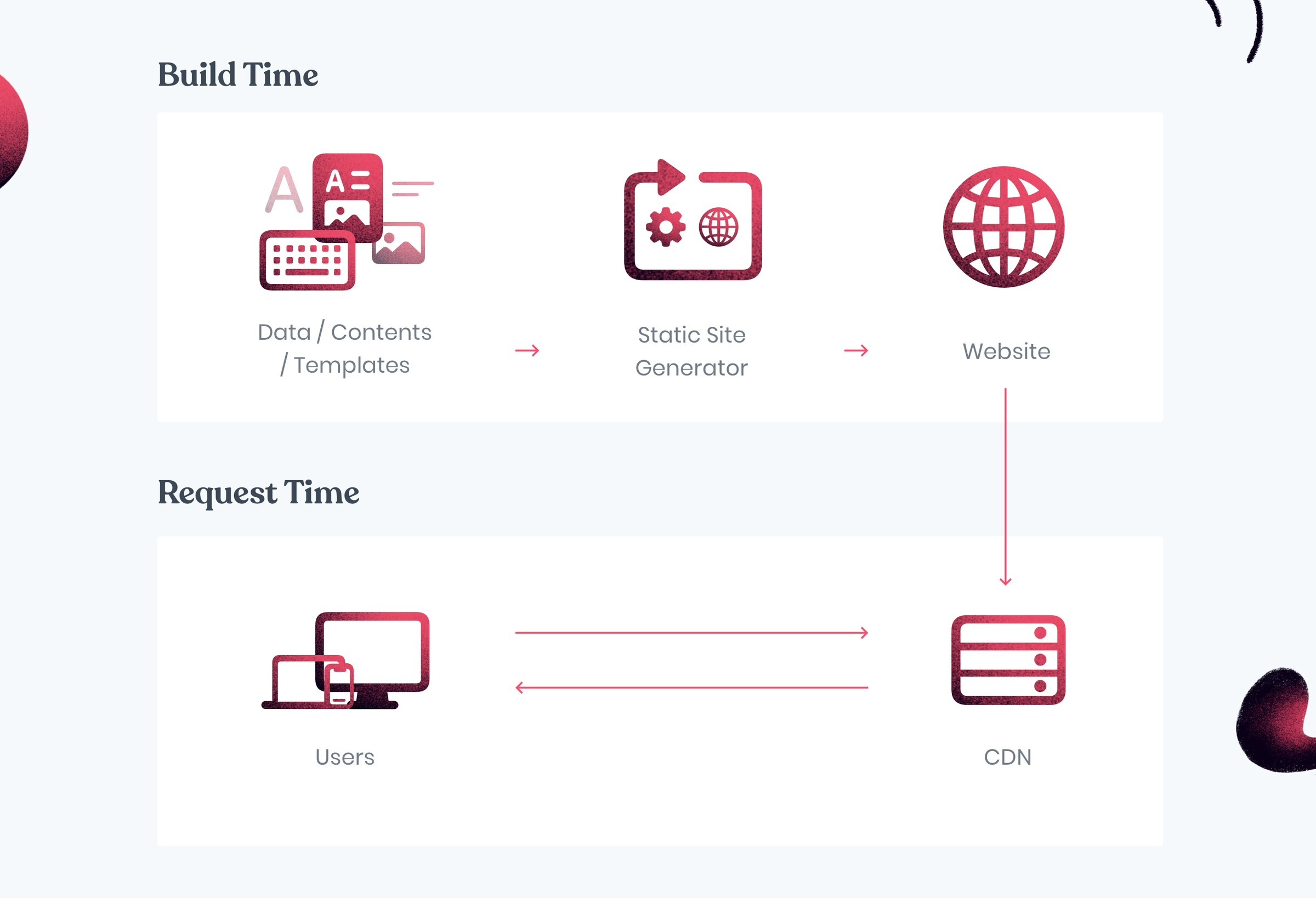
By providing only static files, the potential for harmful attacks is reduced, and security issues are minimized. Additionally, the server-side architecture becomes simpler, making maintenance and scalability easier.
In addition, there are cost benefits to using static site generators. By utilizing a CDN, not only can you achieve stable high-speed performance and constant connectivity globally, but you can also significantly reduce hosting costs.
1-2 Disadvantages of Static Site Generators
Surprisingly, static site generators also have their drawbacks. The workflow is more developer-oriented and significantly different from the traditional monolithic approach, requiring more technical skills to handle SSGs. Additionally, simply using an SSG may be challenging for content managers and editors. Fortunately, the ecosystem of tools focused on static site development is expanding, allowing for easy solutions to this problem through the use of numerous headless CMS solutions.
Adding dynamic elements and user interactions to a page can be tricky, but the Jamstack ecosystem already has solutions for that.
The decision-making process (which SSG to choose) has become unexpectedly difficult due to the very rich selection of options available.
1-3 How to Choose a Static Site Generator?
Choosing the right static site generator depends on the project's needs, the experience of the team involved in the project, the features required for the selected project, and the programming languages used.
1-4 What are we building?
A simple blog for projects is not the same as a feature-rich e-commerce site. Additionally, SSG comes in various "forms," some of which are designed with specific front-ends or back-ends in mind, but that's not all. There are also those created to quickly build photo gallery sites. Furthermore, some make it easier to create documentation pages or websites. Most of them help manage a typical website with a blog.
1-5 Who is it for?
Let's consider who will use/edit the website, taking into account the needs of the project. While it's great that using SSG can improve development efficiency, will non-technical users be working on this site? If so, please think about how to avoid burdening them. Consider the combination of SSG and a headless CMS.
1-6 What languages and frameworks are there?
SSG has evolved significantly in recent years. Nowadays, you can find site generators based on a vast number of programming languages, using various template languages and conventions, that operate in all kinds of environments.
Are you coding with JavaScript? Among the popular SSGs are Next.js and Gatsby. Are you tired of heavy JS frameworks? Check out Eleventy. Do you like writing in Go? In that case, Hugo is the best choice. If Ruby is your preferred language, Jekyll would be a good option. Are you using Vue? Also, try Nuxt.js and Gridsome.
Using your preferred language or framework can make the work easier, but please keep the project's needs in mind first.
What is the most suitable static site generator in 2021?
In today's world, advising the best option for anything can be a double-edged sword. Therefore, instead of a ranking of the "best," we would like to introduce some of the most popular options we have used so far.
To make it easy to understand and compare, the reviews of each static site generator have the same structure. First, we will discuss the history and current status of the project.
Next, we will introduce our excellent features and strengths. After that, we will focus on the ecosystem and showcase some of the most popular projects.
Finally, we will introduce how to start and deploy the project using the SSG in question. And at the end, we will review the best features and usage examples.
2. Next.js
Next.js started as a small framework built on top of React, Webpack, and Babel, designed for server-rendered universal JavaScript web applications without configuration. Initially, it focused on enabling a smooth universal JavaScript experience. It has since evolved to support static site generation, TypeScript, dynamic pages, and serverless functions.
2-1 Next.js 1.0 - The Beginning
I discovered the first version of Next.js in the fall of 2016. I created a simple file like the one below.
// pages/index.js
import React from 'react'
export default () => <div>Hello world!</div>
When I ran it in the terminal, magic happened. With just two lines of code and one command, my new hello world site was up and running. That experience was incomparable to developing a custom theme for WordPress. Next.js and Now (now Vercel) felt incredibly easy.
A few months later, we launched our first project using Next.js and the WordPress API. This project had serious performance issues. Fetching data for each request from many API endpoints was not the best idea. Perhaps we should have exported those pages as static pages. However, in the first version of Next.js, that was not a trivial matter.
Fast Forward to Next.js 9.3
Next.js has evolved to support static site generation. Vercel has evolved to support serverless architecture.
In version 9.3, we introduced three new data acquisition methods.
• getStaticProps: This will be one post content for fetching data at build time.
• getStaticPaths: Specifies a collection of dynamic routes.
• GetServerSideProps: Fetches data for each request.
The following is a simple example of static generation.
// pages/posts/[slug].js
// [slug] filename means it's a dynamic parameter
// it will be passed to getStaticProps `params` object
const BlogPost = ({ data }) => <Post content={data} />
// executed only at build time
export async function getStaticPaths() {
const response = await fetch('https://api.domain/posts')
const data = await response.json()
// all paths we want to pre-render
const paths = posts.map((post) => ({
params: { slug: post.slug }
}))
return { paths }
}
// executed only at build time
export async function getStaticProps({ params }) {
const response = await fetch(`https://api.domain/posts/${params.slug}`)
const data = await response.json()
return { props: { data, fallback: false } }
}
export default BlogPost
In the above code, all blog posts are retrieved from the API at build time, and an array of paths with slugs is created for each. The data for each blog post is fetched in getStaticProps based on the slug parameter.
If you are familiar with Gatsby, you may notice that getStaticProps is somewhat similar to createPages in gatsby-node.js. However, I think Next's approach is clearer. The difference is that you don't need to specify a path to the template and you pass the slug in the context. In Next, everything is contained in the same file. In this case, the value of the slug can be accessed via query parameters.
If you add a new post, you need to rebuild the project unless you change fallback to true. If there are no existing HTML files on the CDN, Next.js will try to fetch and cache the content on the client side. Fallback is very useful when you have a large collection of posts that are frequently updated.
2-3 Image Components
In Next.js 10, particularly, new built-in image components and optimizations have been introduced. From now on, you won't have to worry about shipping large images for mobile devices. Next handles resizing and generates the latest WebP image format, which is about 30% smaller than JPG. If your website has many images, bandwidth and client-side performance will significantly decrease.
<Image /> To use the component, please import it from next/image.
import Image from 'next/image'
const Hero = () => (
<section>
<Image src="/assets/cute-hero.png" width={500} height={500} />
<h1>Hello world!</h1>
</section>
)
export default Hero
The approach of Next.js has no impact on build time. All optimizations are performed at request time. Currently, Next supports four cloud providers: Vercel, Imgix, Cloudinary, and Akamai.
2-4 File Structure
Next.js is zero-config from the start.
├── package-lock.json
├── package.json
├── pages
│ ├── about.js
│ ├── blog
│ │ ├── [slug].js
│ │ └── index.js
│ └── api
│ ├── posts.js
│ ├── about.js
│ ├── blog.js
│ ├── contact.js
│ └── index.js
└── public
└── logo.png
All files in the src/api directory are lambda functions.
2-5 Ecosystem
Next.js has a wonderful community that continues to grow. You can read interesting conversations and discuss RFCs on Github. The Vercel team is clarifying the direction of the framework and is open to suggestions from the community.
There are many examples demonstrating integration with various tools such as Headless CMS, CSS-in-JS, and auth.
2-6 How to Get Started?
The easiest and recommended way to start a new Next.js project is to use create-next-app.
npx create-next-app
# or
yarn create next-app
With this one command, you will set up the project. For those who want to know more, we recommend the official Learn Next tutorial.
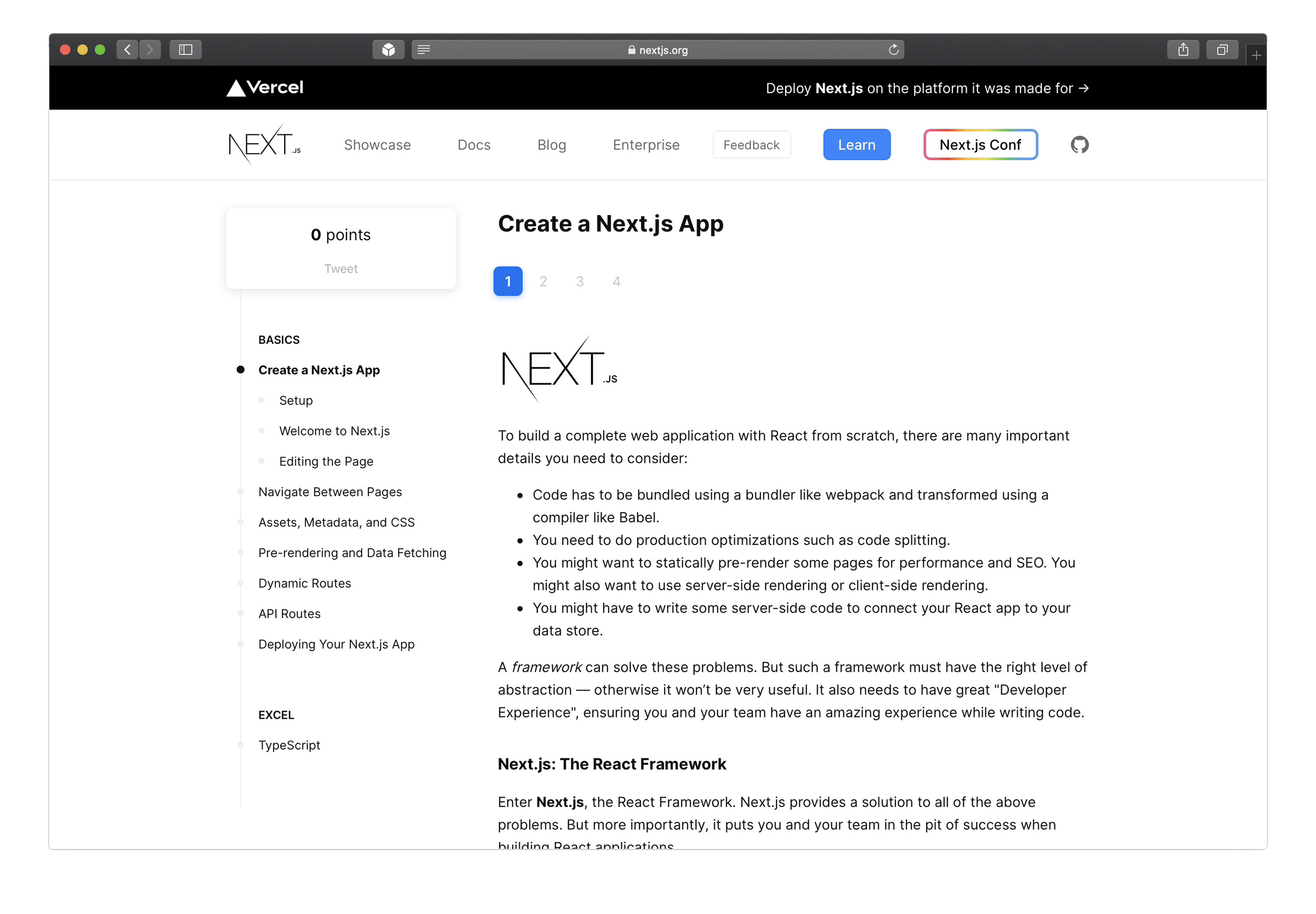
It helps you understand how to navigate between pages, add static assets, and fetch data. Once this is complete, you will be ready to build your first Next.js application.
2-7 Next.js Deployment
The recommended platform is, of course, Vercel. Their CDN is designed for the edge and supports features like incremental static generation. First, the page is input into a durable store (S3), and then the pathname is purged so that users can see the latest content.
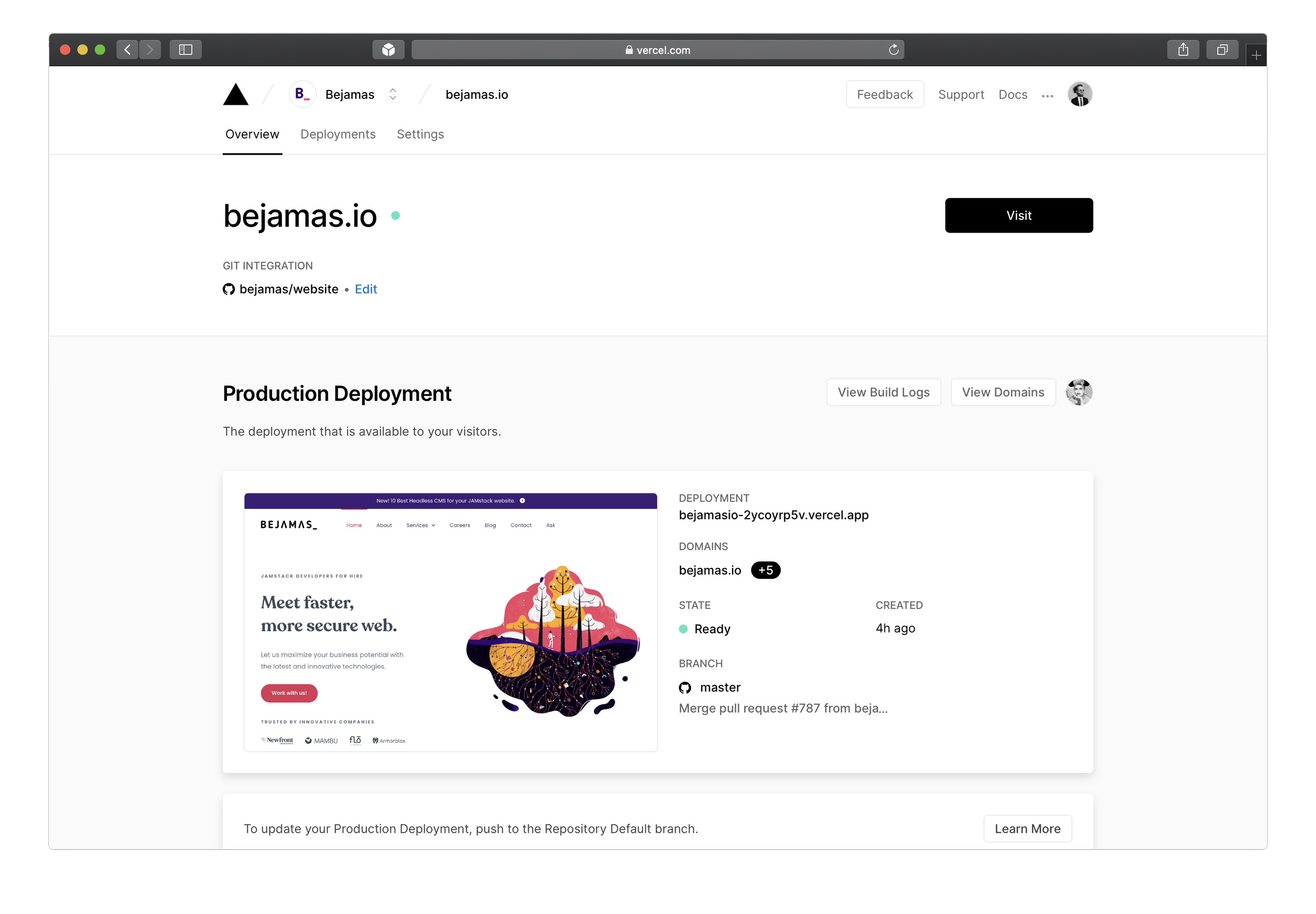
Preview mode works seamlessly on the Vercel platform, just like the fallback feature. Once you connect the repository, everything works immediately without any additional configuration.
If you do not want to use Vercel for any reason, you can implement Next.js on any modern hosting platform such as Netlify, Render, AWS, or DigitalOcean. Netlify manages a special next-on-netlify package that enables server-side rendering of pages. Features like static image differential playback, fallback, and preview do not function exactly the same as Vercel, so it is not a one-to-one replacement.
2-8 Conclusion
With Next.js, you can build all kinds of websites and applications, from simple marketing pages and blogs to e-commerce and PWAs using cookie-based authentication.
Ideal for projects that require flexible construction of specific parts of a website. The first static site generator with dynamic extensions. The hybrid mode is truly amazing. If you don't need React and don't plan to update the project frequently, you may not need something this advanced.
- Zero Configuration
- Demonstrates excellent experiential performance through instant route changes and prefetching
- Support for TylieScrilit
- Automatic Code Splitting
- Dynamic API Route
- The Vercel team is always working to reduce JS bundle size and optimize performance
- Integrating efforts with the new Web Vitals metrics, we will analyze Next.js
- Hybrid Mode: Both Static Generation and SSR
- Built-in image components and on-demand image optimization
- Top-notch support for internationalization
- Hybrid app. When both static pages and server-side rendered pages are needed for each request.
- E-commerce. Especially when there is high traffic and frequent content updates.
- Landing pages and marketing sites, especially those already using React and design systems
- Website utilizing interactive React components
- A site with many advanced route transitions